Battle-Tested Strategies for Scaling Cloud-Native Applications
Cloud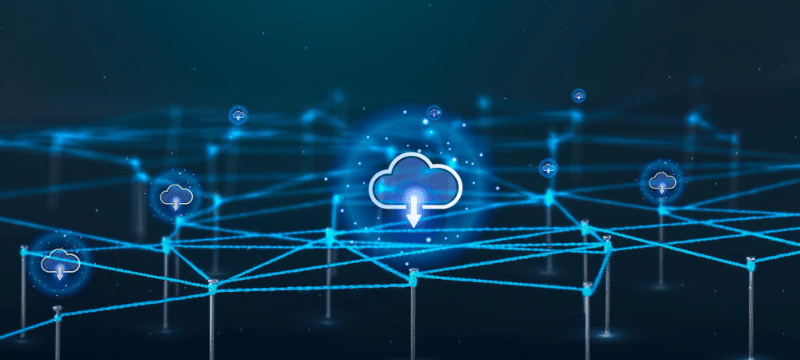
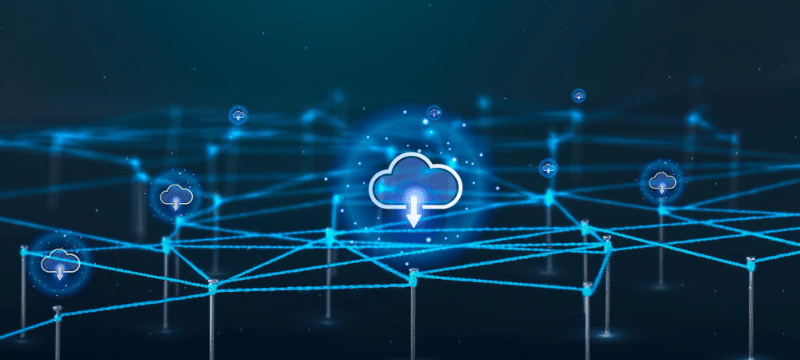
Every engineer who’s watched their application crumble under unexpected load knows the harsh truth: Scaling is more than just adding more servers. We’ve seen carefully designed microservice architectures fall apart during high-demand periods like Black Friday sales and product launches—not because of poor application code, but because the organization hadn’t implemented the right patterns for scalability of the cluster or the application.
Proper scaling requires thoughtful architecture that anticipates surges and implements the right resilience patterns before they’re needed. Here, we’ll share proven scaling techniques that have been implemented across dozens of containerized applications running on Kubernetes or other orchestration platforms. Our examples come from real projects, from startup MVPs to enterprise systems processing millions of requests every minute. Instead of theoretical concepts from certification courses, we’re giving you practical approaches with specific configuration examples you can apply to your clusters today.
Fine-tuning Kubernetes autoscaling for application performance
Before anything else, when you are getting started with containers on your cloud server, optimize your container orchestration ecosystem. This first step ensures both better performance and lower costs for your cloud-native applications. By default, a basic Kubernetes deployment won’t automatically scale with changing workloads. This leaves your application vulnerable to performance degradation during traffic spikes or unnecessary resource consumption during low-demand periods.
To enable systems that expand and contract with almost organic fluidity, maintaining performance while eliminating waste during leaner periods, consider one or a combination of the following scaling strategies:
Horizontal Pod Autoscaler
The Horizontal Pod Autoscaler (HPA) adjusts the number of pod replicas in a deployment, replication controller, or replica set based on CPU usage or custom metrics.
To implement an HPA, first ensure the metrics server is installed in your cluster:
kubectl apply -f https://github.com/kubernetes-sigs/metrics-server/releases/latest/download/components.yaml
Then create an HPA using the kubectl command:
kubectl autoscale deployment darwin-app --cpu-percent=80 --min=2 --max=10
The above command creates an autoscaler that maintains between 2 and 10 replicas of the pods controlled by the darwin-app deployment. The HPA will scale replicas to maintain an average CPU utilization of 80% across all pods.
Alternatively, you can define an HPA using a YAML manifest:
apiVersion: autoscaling/v2
kind: HorizontalPodAutoscaler
metadata:
name: darwin-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: darwin-app
minReplicas: 2
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 80
One of the beauties of HPA is that it allows scaling based on custom metrics, not just CPU and memory. For example, you can scale based on requests-per-second or queue length by configuring a custom metrics adapter.
Vertical Pod Autoscaler
While HPA scales by changing the number of pods, the Vertical Pod Autoscaler (VPA) adjusts the CPU and memory requests/limits of containers within pods.
To implement VPA, first install it in your cluster:
git clone https://github.com/kubernetes/autoscaler.git
cd autoscaler/vertical-pod-autoscaler/
./hack/vpa-up.sh
Then create a VPA resource pointing to your deployment:
apiVersion: autoscaling.k8s.io/v1
kind: VerticalPodAutoscaler
metadata:
name: darwin-app-vpa
spec:
targetRef:
apiVersion: "apps/v1"
kind: Deployment
name: darwin-app
updatePolicy:
updateMode: "Auto"
resourcePolicy:
containerPolicies:
- containerName: '*'
minAllowed:
cpu: 100m
memory: 50Mi
maxAllowed:
cpu: 1
memory: 500Mi
controlledResources: ["cpu", "memory"]
This VPA will automatically adjust the resource requests for containers in the darwin-app deployment between the specified minimum and maximum values.
Cluster Autoscaler
The Cluster Autoscaler adjusts the size of your Kubernetes cluster when pods fail to schedule due to resource constraints or when nodes are underutilized.
To implement the Cluster Autoscaler on your cloud server:
apiVersion: apps/v1
kind: Deployment
metadata:
name: cluster-autoscaler
namespace: kube-system
labels:
app: cluster-autoscaler
spec:
replicas: 1
selector:
matchLabels:
app: cluster-autoscaler
template:
metadata:
labels:
app: cluster-autoscaler
spec:
serviceAccountName: cluster-autoscaler
containers:
- image: k8s.gcr.io/autoscaling/cluster-autoscaler:v1.22.0
name: cluster-autoscaler
resources:
limits:
cpu: 100m
memory: 300Mi
requests:
cpu: 100m
memory: 300Mi
command:
- ./cluster-autoscaler
- --v=4
- --stderrthreshold=info
- --cloud-provider=DRW
- --skip-nodes-with-local-storage=false
- --expander=least-waste
- --node-group-auto-discovery=asg:tag=k8s.io/cluster-autoscaler/enabled,k8s.io/cluster-autoscaler/my-cluster
env:
- name: DRW_REGION
value: us-west-2
The Cluster Autoscaler proactively monitors for pods that fail to schedule and for underutilized nodes. When pods can’t be scheduled, it increases the size of the node group. When nodes are underutilized for an extended period, it removes them from the cluster.
Multidimensional scaling
For optimal resource utilization and application performance, it’s highly recommended to implement all three scaling mechanisms together to reap the benefits of both horizontal and vertical scaling:
- HPA adjusts the pod numbers based on workload
- VPA ensures each pod has the right resource allocation
- Cluster Autoscaler makes sure the underlying infrastructure scales appropriately
When properly configured, these three scaling mechanisms work together to ensure your application scales efficiently in response to changing workloads.
For example, during a traffic spike:
- HPA will increase the number of pods
- If the pods can’t be scheduled due to insufficient cluster resources, Cluster Autoscaler will add more nodes
- Meanwhile, VPA will continuously optimize the resource requests of your containers
Advanced scaling patterns for complex cases
If you are building modern distributed systems, you’ll eventually encounter situations where basic scaling approaches prove insufficient. As your system’s architecture becomes more complex, you’ll need to use more sophisticated diagonal scaling patterns to handle the unique challenges of cloud-native environments.
Predictive auto-scaling
Very frequently, reactive scaling introduces latency during traffic spikes as the system detects load increases and provisions new resources. Predictive auto-scaling solves this problem by anticipating demand before it occurs.
Implement machine learning that studies your past traffic patterns. This helps spot connections between usage spikes and specific times, seasons, or business events. When clear patterns emerge, your system can automatically prepare extra resources before the expected traffic arrives.
Businesses with cyclical workloads typically see substantial benefits from this approach. If you are operating e-commerce platforms, streaming services, or business applications with predictable usage patterns, predictive scaling would provide a better user experience while optimizing resource utilization.
Circuit breaking and bulkheading
When your services start failing, one slow service can make your entire app crash. Circuit breakers act like automatic fuses in your code to prevent this.
In your next implementation, add circuit breakers around external API calls and database operations. Libraries like Resilience4j make this straightforward. Just wrap your service calls and configure thresholds like “trip after 5 failures in 10 seconds.” When a circuit trips, your code can immediately fall back to a backup plan instead of waiting for timeouts.
One e-commerce client recently saved their checkout flow during a payment provider outage by implementing circuit breakers with a simple fallback that put orders into a processing queue when direct payment calls failed.
For better isolation, use bulkheading to give critical services their own resources. It’s a fool-proof, practical way of moving your payment processing (or similar critical apps) to a dedicated connection pool or separate container with guaranteed resources. When your recommendation engine starts hogging CPU, your checkout service stays responsive because it’s protected in its own “compartment.” Most cloud platforms now make this easy with resource quotas and service isolation.
Spot instances and preemptible VMs
Workloads capable of withstanding occasional interruptions present excellent candidates for discount compute options like spot instances or preemptible VMs. The financial advantage proves considerable, essentially on account of discounts that typically range from 60-90% compared to standard rates.
The success factor is choosing architectural designs that smoothly handle instance termination and replacement. This strategy works remarkably well for stateless components, batch processing jobs, and development environments.
For advanced implementations, you can also employ hybrid approaches that maintain core capacity with standard instances while expanding with opportunity-based spot resources. This balanced strategy helps achieve significant cost reductions while preserving system reliability.
Performance tuning for distributed systems
Distributed architectures introduce performance considerations that differ substantially from monolithic systems. Optimizing these environments demands specialized approaches focused on communication efficiency and resource coordination.
Data locality optimization
The physical distance between data and compute resources is one of the most critical constraints in distributed systems. To minimize unnecessary data movement across network boundaries, it is recommended to adopt effective locality strategies.
Consider leveraging techniques such as multi-tier caching, access-pattern-aligned data partitioning, and strategic service placement to reduce network traversal. Careful data placement often boosts performance more than code optimization.
Connection pooling and backpressure
Every time your application creates a new database connection, it wastes precious milliseconds on handshakes and authentication. This overhead adds up quickly in high-traffic systems. Connection pooling solves this by keeping a set of pre-established connections ready to use, dramatically cutting request latency.
In practice, you’ll want to configure your connection pools carefully. Set minimum and maximum pool sizes based on your workload patterns. Too small and requests queue up waiting for connections, too large and you waste resources on idle connections.
When your system gets overwhelmed, backpressure mechanisms act as your safety valve. Instead of crashing under heavy load, components can signal they’re reaching capacity limits. This might mean temporarily queuing lower-priority requests or returning a “try again later” response, keeping critical functions running while the system catches up. Many modern frameworks include these patterns, but you’ll need to enable and tune them for your specific needs.
Final thoughts
Throughout our journey of exploring cloud-native scalability, we’ve observed numerous organizations achieving remarkable success with properly implemented scaling strategies. Conversely, we’ve witnessed teams struggling with performance bottlenecks and unexpected expenses when applying one-size-fits-all approaches.
At Kamatera, we’ve learned that the most successful cloud native implementations come from teams that view scaling techniques as part of a broader architectural toolkit. Rather than reflexively applying auto-scaling to every component, these organizations carefully analyze workload patterns and select the most appropriate scaling approach for each specific service.
As cloud native technologies continue to mature, many of today’s scaling challenges will be addressed through improved tooling and practices.